Extract cwt
1import numpy as np
2import audioflux as af
3from audioflux.type import SpectralFilterBankScaleType, WaveletContinueType
4
5# Get a 880Hz's audio file path
6sample_path = af.utils.sample_path('880')
7
8# Read audio data and sample rate
9audio_arr, sr = af.read(sample_path)
10
11# Create CWT object and extract cwt
12cwt_obj = af.CWT(num=84, radix2_exp=12, samplate=sr,
13 wavelet_type=WaveletContinueType.MORSE,
14 scale_type=SpectralFilterBankScaleType.OCTAVE)
15
16# The cwt() method can only extract data of fft_length=2**radix2_exp=4096
17cwt_arr = cwt_obj.cwt(audio_arr[..., :4096])
18cwt_arr = np.abs(cwt_arr)
19
20# Display spectrogram
21import matplotlib.pyplot as plt
22from audioflux.display import fill_spec
23audio_len = audio_arr.shape[-1]
24fig, ax = plt.subplots()
25img = fill_spec(cwt_arr, axes=ax,
26 x_coords=cwt_obj.x_coords(),
27 y_coords=cwt_obj.y_coords(),
28 x_axis='time', y_axis='log',
29 title='CWT Spectrogram')
30fig.colorbar(img, ax=ax)
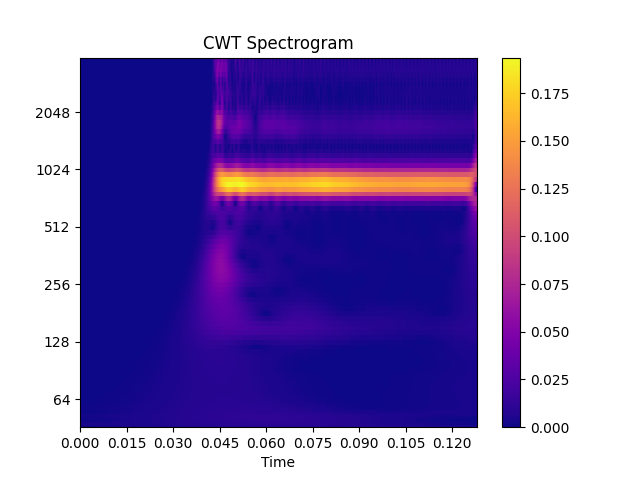